Introduction:
This article explains, - How to read the XML using LINQ
- How to update the XML element values using LINQ
Explanation:
Below namespace needs to added in order to work using LINQ
using System.IO;
using System.Linq;
using System.Xml;
using System.Xml.Linq;
Sample XML String:
private const string XmlValue = @"<RootNode>
<Item>
<Key>ItemKey1</Key>
<Value>1</Value>
</Item>
<Item>
<Key>ItemKey2</Key>
<Value>2</Value>
</Item>
<Item>
<Key>ItemKey3</Key>
<Value>3</Value>
</Item>
<Item>
<Key>ItemKey4</Key>
<Value>4</Value>
</Item>
<Item>
<Key>ItemKey5</Key>
<Value />
</Item>
<Item>
<Key>ItemKey6</Key>
<Value>6</Value>
</Item></RootNode>";
Read and Update the XML string:
Here I have used StringReader and
XML Reader to read the XML string.
In this case, XmlReader is used
to read the XML string. In case of XML file, XDocument will work with
XMLReader.
XMLReader has below overload
methods to read the XML String:
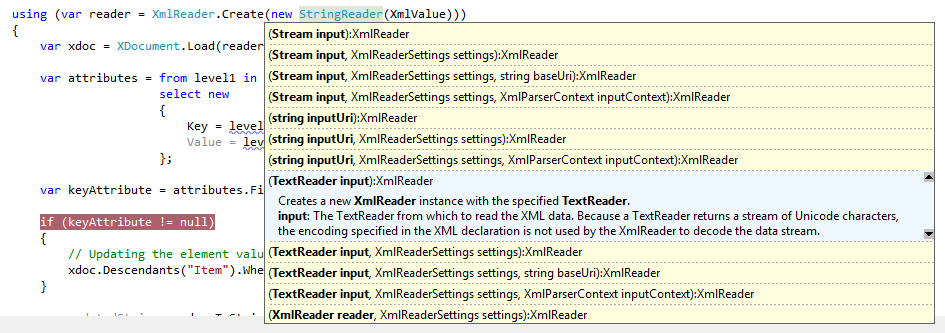
XDocument has below overload
methods to load the XML values:
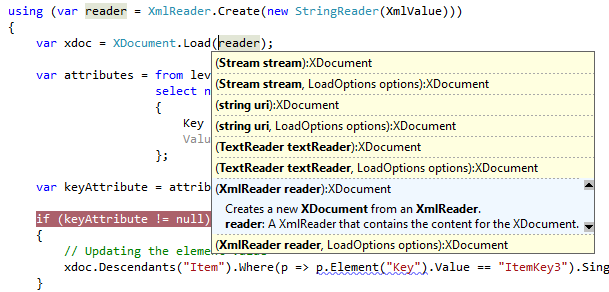
Below code explains how to work
with XmlReader and XDocument using LINQ
// Reading the XML string using XmlReader
to pass to XDocument.
using (var reader = XmlReader.Create(new
StringReader(XmlValue)))
{
// Loads the XDocument
var xdoc = XDocument.Load(reader);
// Using LINQ Query to find the items in the
XML.
var attributes = from level1 in xdoc.Descendants("Item")
select new
{
Key =
level1.Element("Key").Value,
Value =
level1.Element("Value").Value
};
// Finding the keys/search values
var keyAttribute =
attributes.FirstOrDefault(p => p.Key == "ItemKey3");
if (keyAttribute != null)
{
// Updating the element value directly on the
XML string.
xdoc.Descendants("Item").Where(p
=> p.Element("Key").Value == "ItemKey3").Single().SetElementValue("Value", "1000");
}
var updatedString = xdoc.ToString(); // This has the updated XML string.
// Save the document in the desired path.
xdoc.Save(@"D:\\updatedXML.xml");
} |