WCF Extensibility supports validating the request before
reaching the corresponding endpoints. It is explained in simpler way below with
sample code.
1. Understanding
“IParameterInspector” Interface:
ü
“IParameterInspector” resides in the System.ServiceModel.Dispatcher
interface.
ü
WCF can easily validate the input parameters and
its outgoing response by implementing “IParameterInspector” interface.
ü
“IParameterInspector”
interface having two below methods which will help us to inspect the request
and response.
o
BeforeCall - It will be called before hitting the
endpoint
o
AfterCall –
It will be called before sending response to Client.
After implementing the “BeforeCall ” & “AfterCall” methods from the “IParameterInspector”
interface , the corresponding class needs to be added in the OperationBehavior so that the
inspection will happen before reaching the endpoints.
2. Understanding
“IOperationBehavior” Interface:
ü
Class which implemented “IParameterInspector” needs to be
added in “IOperationBehavior”
implemented class to intercept the request to do request validation.
Above mentioned “IParameterInspector” and “IOperationBehavior”
implementation is explained in easy steps below.
3. “IParameterInspector”
Implementation:
public class ValidateInputParameters : IParameterInspector
{
int index = 0;
public ValidateInputParameters()
: this(0)
{ }
public ValidateInputParameters(int index)
{
this.index = index;
}
#region
IParameterInspector Member Implementation
public void AfterCall(string operationName, object[] outputs, object returnValue, object correlationState)
{
}
public object BeforeCall(string operationName, object[] inputs)
{
if (operationName == "AddTwoIntegers")
{
if (inputs.Count() != 2)
{
throw new FaultException("Enter two integers which are greater than
zero.");
}
int i = 0;
if (!int.TryParse(inputs[0].ToString(), out i) || !int.TryParse(inputs[0].ToString(),
out i))
{
throw new FaultException("Enter input values as integers.");
}
if (Convert.ToInt32(inputs[0]) <= 0 || Convert.ToInt32(inputs[1])
<= 0)
{
throw new FaultException("Both input numbers should be greater than
zero.");
}
}
return null;
}
#endregion
}
4. “IOperationBehavior”
Implementation:
public class InputParameterValidation : Attribute, IOperationBehavior
{
int index = 0;
public InputParameterValidation() : this(0) { }
public InputParameterValidation(int index)
{
this.index = index;
}
#region
IOperationBehavior Implementation
public void AddBindingParameters(OperationDescription operationDescription, System.ServiceModel.Channels.BindingParameterCollection
bindingParameters)
{
}
public void ApplyClientBehavior(OperationDescription operationDescription, System.ServiceModel.Dispatcher.ClientOperation
clientOperation)
{
clientOperation.ParameterInspectors.Add(new ValidateInputParameters(index));
}
public void ApplyDispatchBehavior(OperationDescription operationDescription, System.ServiceModel.Dispatcher.DispatchOperation
dispatchOperation)
{
dispatchOperation.ParameterInspectors.Add(new ValidateInputParameters(index));
}
public void Validate(OperationDescription operationDescription)
{
}
#endregion
}
5. Decorate
OperationContract with InputParameterValidation:
[ServiceContract]
public interface IService1
{
[InputParameterValidation]
[OperationContract]
string AddTwoIntegers(int number1, int number2);
}
By doing so, the request will trigger the “BeforeCall”
method implemented in the “ValidateInputParameters”
class and validation will occur on the input parameters.
Testing the
OperationContract:
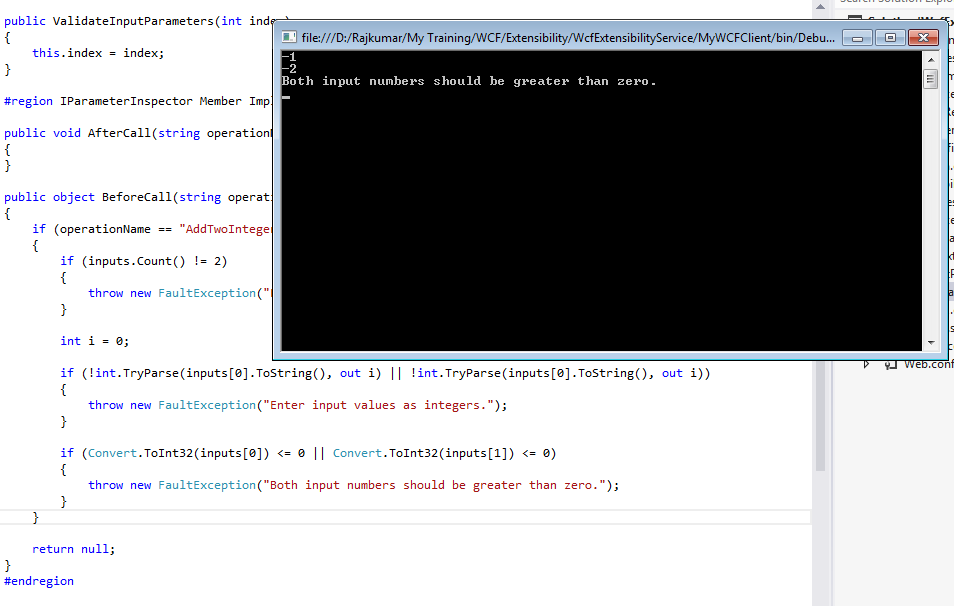
|