Introduction
This demo and diagrammatical explanation mainly targets the
.Net developers who are total beginners in the web application world and want
to get started on familiar Microsoft .Net technologies.
Prerequisites
- Html basics – This is
needed to program the user interface as there are no controls to drag and drop
components like seen in WPF applications.
- CSS basics – This is not a
mandatory skill that’s needed, but can be useful to give a good finishing touch
to the web page making it look more professional.
- C# - You should be very
comfortable working with this language as this is the flesh and blood of the
application.
Development Environment
I will be using Visual studio 2012 Ultimate Edition for all
demonstrations. In case the application you are using is a lower version such
as Visual studio 2010 then you will have to download the ASP.NET MVC 4 from the
Microsoft download center. Do this by visiting the below link.
http://www.microsoft.com/en-in/download/details.aspx?id=30683
MVC – What exactly is it!?
MVC stands for Model, View and Controller. It is not a
Microsoft technology! It’s a common design pattern that exists in many web
application frameworks such as Ruby on Rails, Django and Zend. MVC is commonly
used to structure user-oriented applications.
Model
It represents the structure of your data that you want to
make visible to the user. It’s a plain vanilla class that defines properties.
Model is intended for the view and not necessarily the entire application.
View
View is the result that the user will see when interacting
with our Web Application. This is typically an HTML page, but it can also be
raw data represented by XML or JSON, or binary data.
Controller
This is a class that performs the necessary linkage between
the View and Model. Each controller exposes a different public method known as Actions. This will be the applications
URL. Each Action in a controller can have a View if needed. Do not confuse a
controller as a business logic layer; all it should do is retrieve data from
the view and vice versa. The data manipulation and processing should be done in
a separate layer.
Routing
It is the process responsible for resolving, or routing a
URL into a specific Action in a specific Controller. It also decides how to
pass parameters for Actions in a particular Controller.
URL: http://www.[DomainName].com/{ControllerName}/{Action}/9
The number 9 is the parameter that is passed to the action. The
RouteConfig.cs file is present in the
App_Start folder of the application.
The controller and the action name can be changed as your wish and thus this
will be the default page that opens up on loading the domain URL. 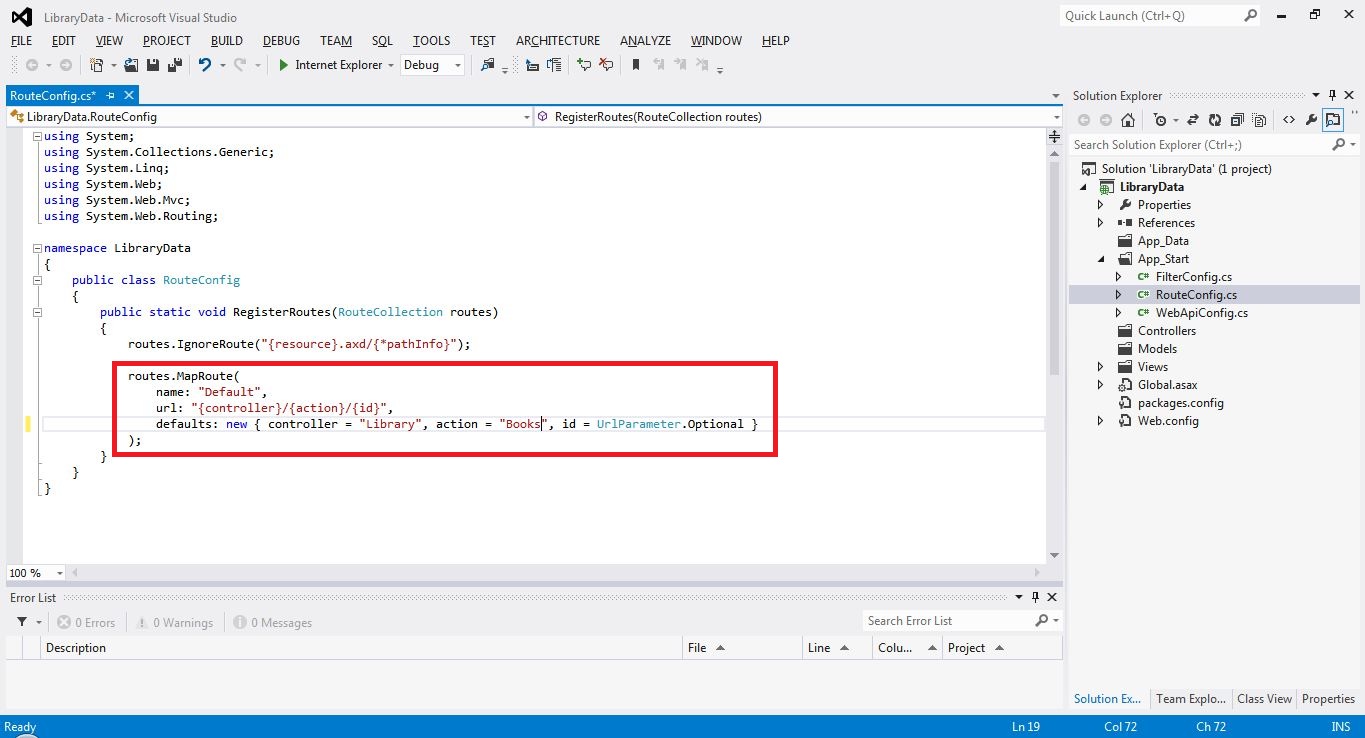
Fig 1 : Shows the default controller name and the action name changed.
The id parameter shown in the URL is optional since it’s defined
as UrlParameter.Optional.
Your First ASP.NET MVC application –
Library Manager Let us try creating an application that manages the quantity
and price of the books coming into the library and make the librarians life
easier. To create a new ASP .NET MVC 4 Application, you will need to create a
new project. In the pop up window, select the Dot Net framework that you intend
to work on and then select the ASP.NET MVC 4 Web Application. I have used 4.5
Framework in this demonstration.
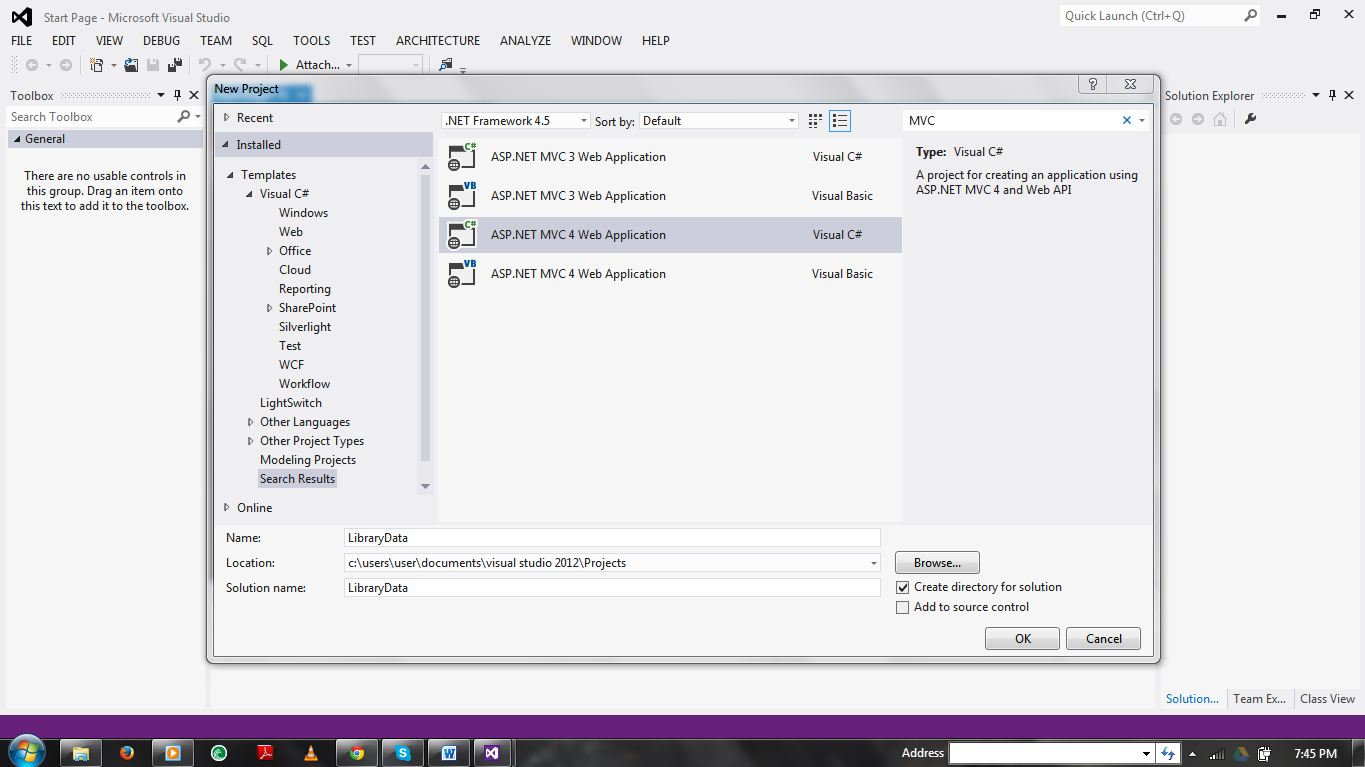
Fig 2: Startup page while creating the MVC 4 project.
Let us name this project as LibraryData and select the
required location to save the project. Once this is done move on to the next
screen by clicking on OK.
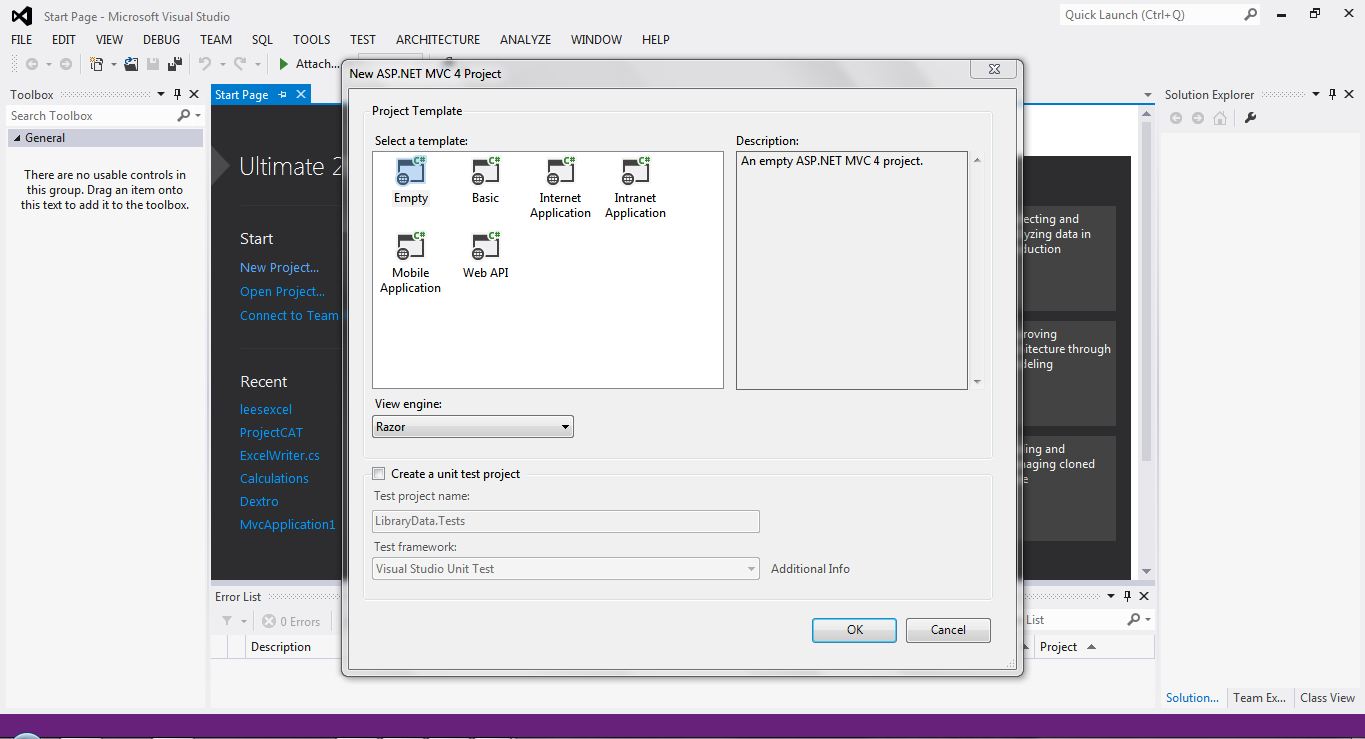
Fig 3: Select empty web application
Make sure to select an empty web application as we are going
to start from the scratch. On clicking ok our application is ready and we are
set to go.
Creating Model
Since the model is the backbone of our application, let’s
start off with creating a simple model class. Name this model class as library. Place this class file in the
Models folder.
Fig 4: Model class Library that is present in the Models folder
Creating the
Controller
Once the model is created, we create the controller by right
clicking the Controller folder and adding the controller. Select an empty MVC
controller.
Fig 5: Adding a controller
Fig 6: Select Empty MVC controller in the Template section
Once the controller is created we can write different
Actions inside. The below diagram demonstrates the Books Action method created in the LibraryController.
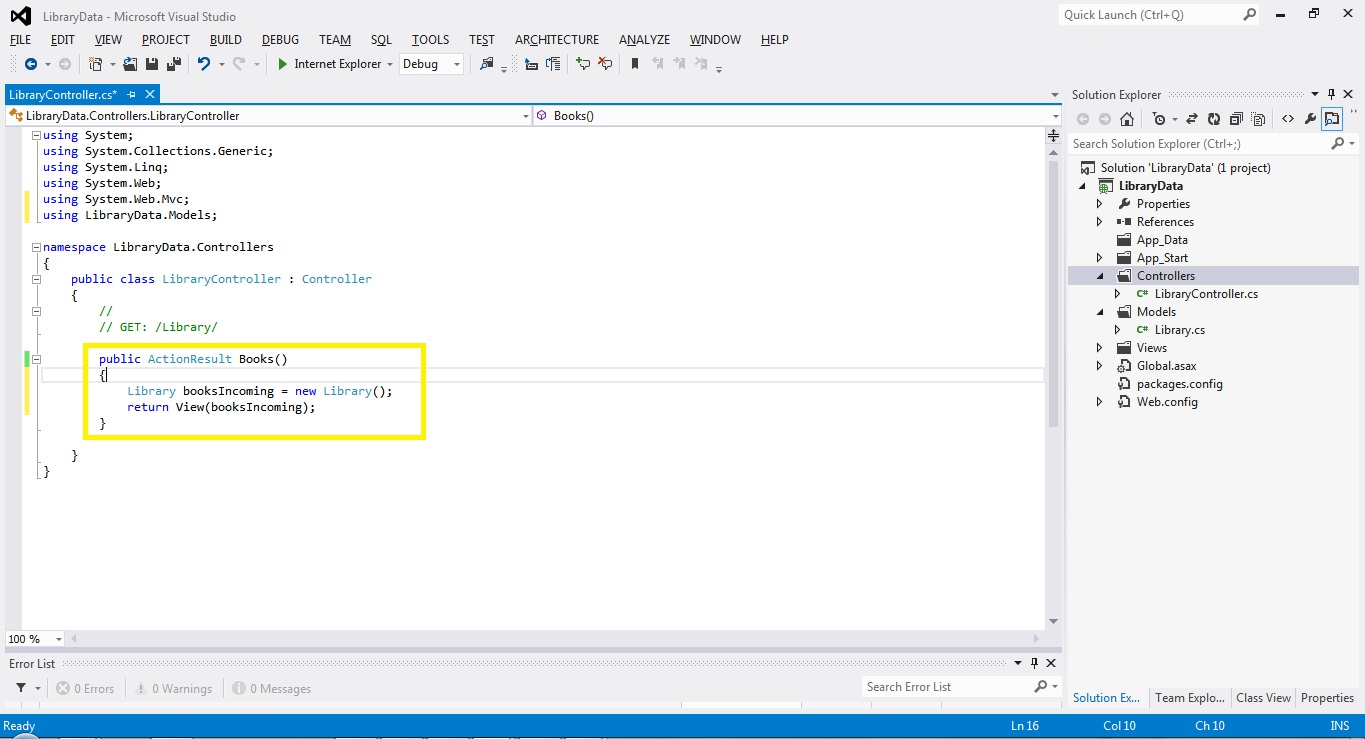
Fig 7: Action inside the
LibraryController
Creating a View
Once the Action method is created the next step to follow is
to create the user interface for that Action. This can be created by right
clicking the action and adding view.
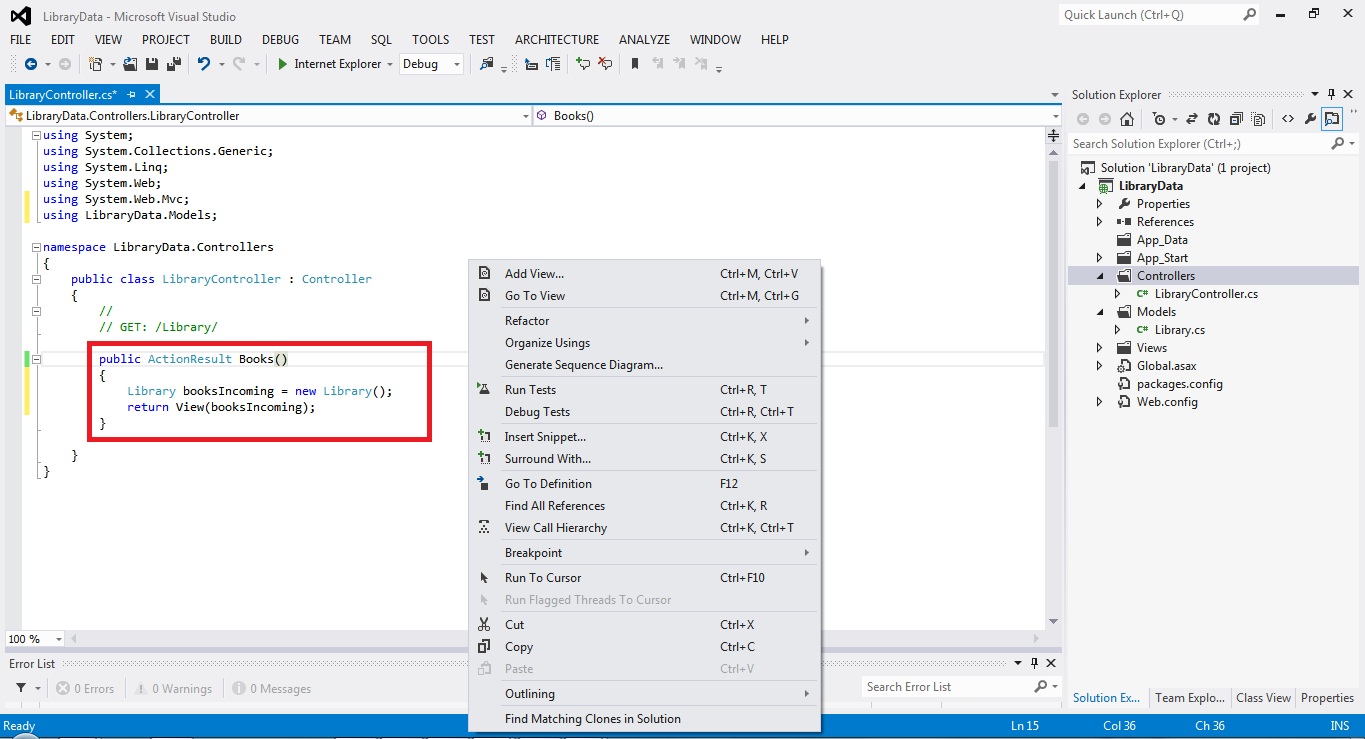
Fig 8: Addition of View
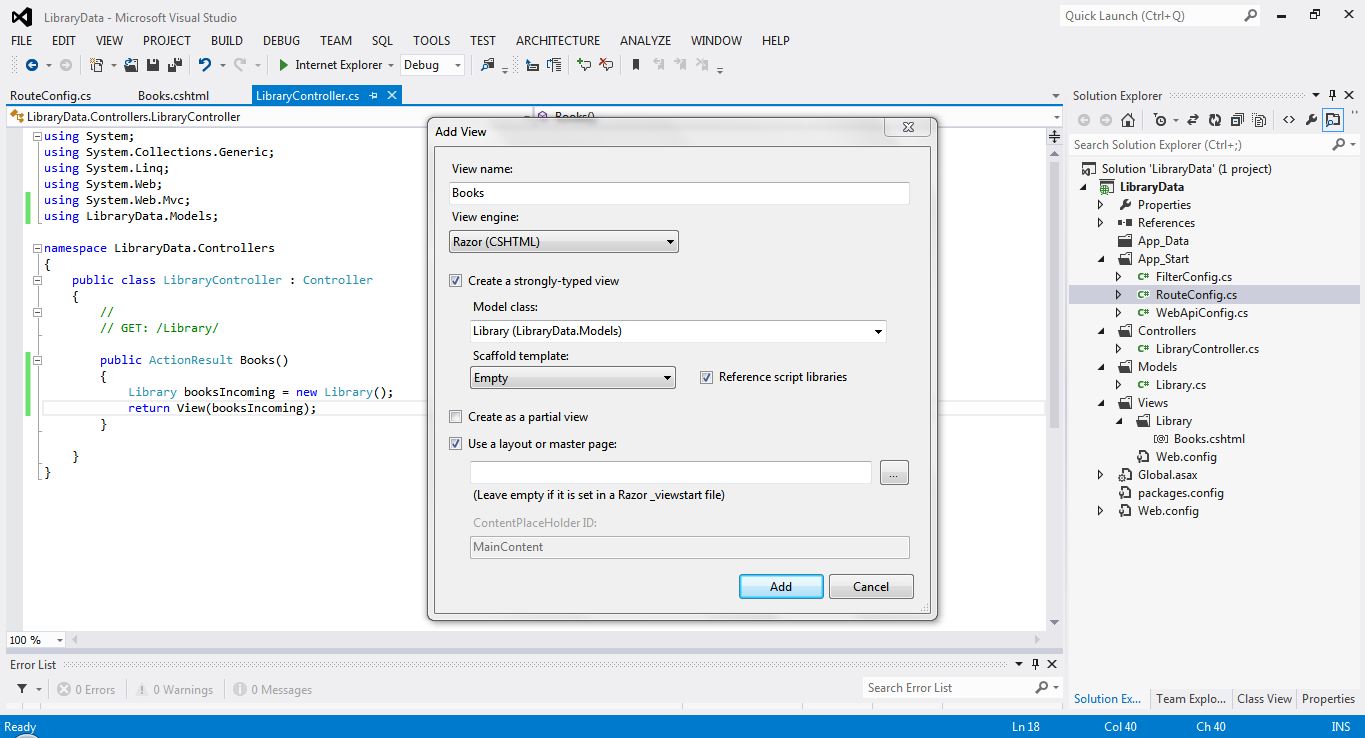
Fig 9: Shows a strongly typed view being created
Let’s create a strongly typed view. For this select the
model class that was created in the Models folder. Select ADD, this creates a
basic structure of a View.
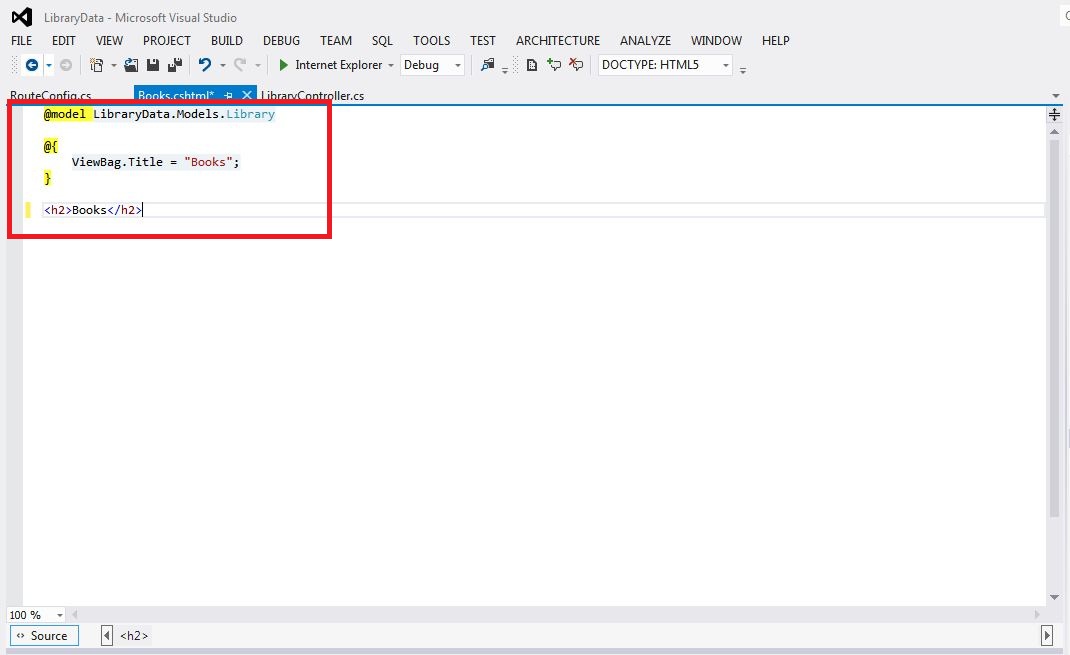
Fig 10: Basic outline of a View
Here the @model is
a keyword reserved by Razor, and states the current Model type associated with
this View. You can then access the instance of this Model type by using the
Model keyword. Notice that capitalization, as usual with C#,
matters. Model with a capital M means a call to the instance of the Model,
and model represents the declaration. Model is strongly typed,
and you can now access the properties of your model class by simply stating the
property name. We are injecting the library class instance to the View from the
Action method in the controller as seen earlier in Fig 9.
Let’s now create a
basic architecture of the view so that it looks a bit presentable.
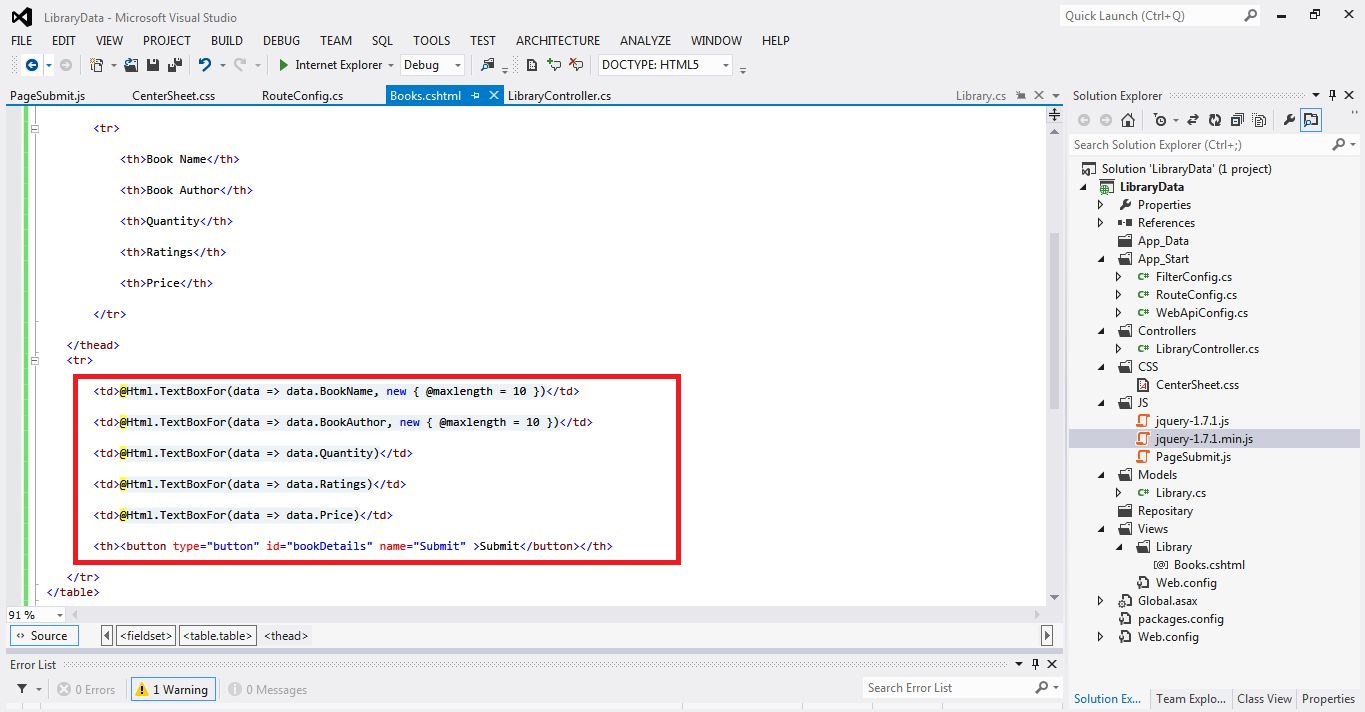
Fig 11: Shows the instance of the model being used
This basically forms a view as shown in Fig 13. The
submit button in the page can be configured to navigate to the action method BookSubmitted using an Ajax post or a
form submit logic.
Fig 12: Form post to Action method Library
The above code
present in the View is used to navigate to the BookSubmitted Action Method in
the Library controller.
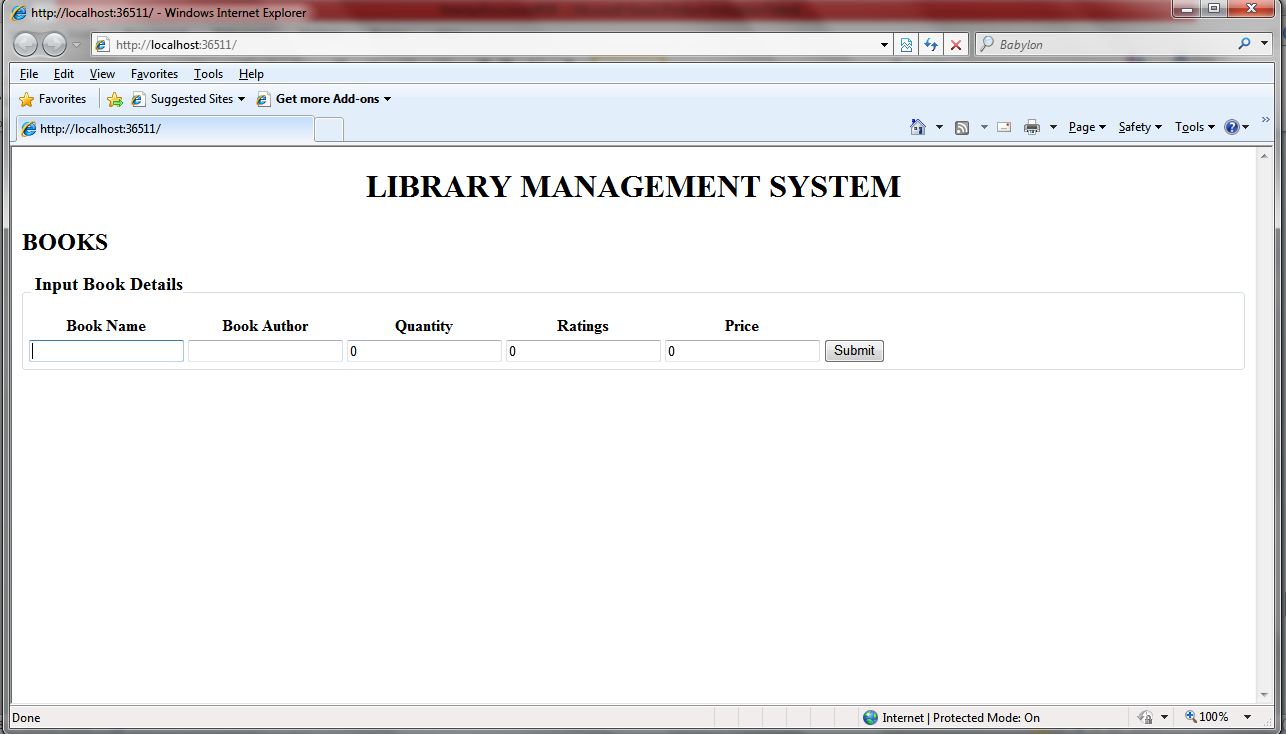
Fig 13: Landing Screen of the application
Once the data is entered and on pressing submit we reach the
Action method called BookSubmitted that we create to get the data inputted in
the UI. This method can be used to navigate the data to the back end database
after the needed manipulation of the data in the business layer.
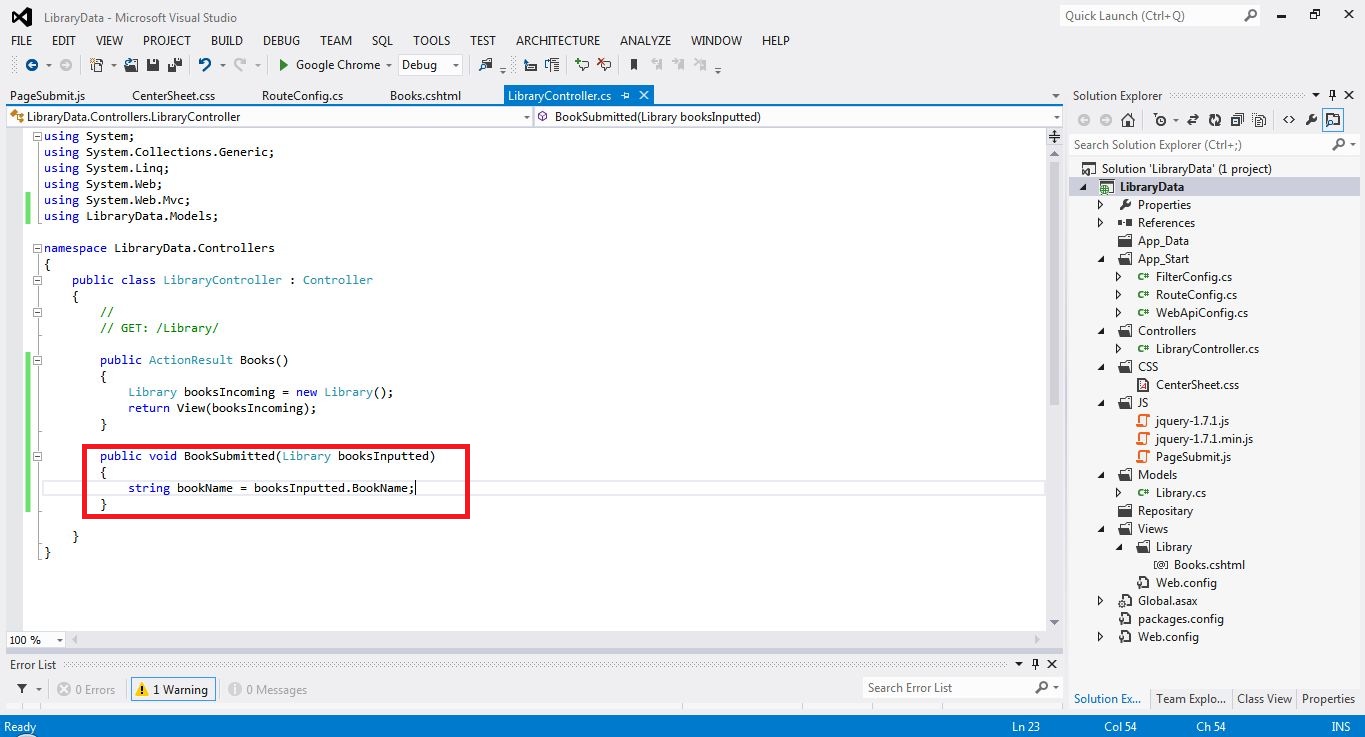
Fig 14: Shows the method where the data from the UI is posted back
Similarly to obtain the data from the database call a
business layer before calling the view, this can obtain the Library books data
and send that parameter to the View to be displayed.
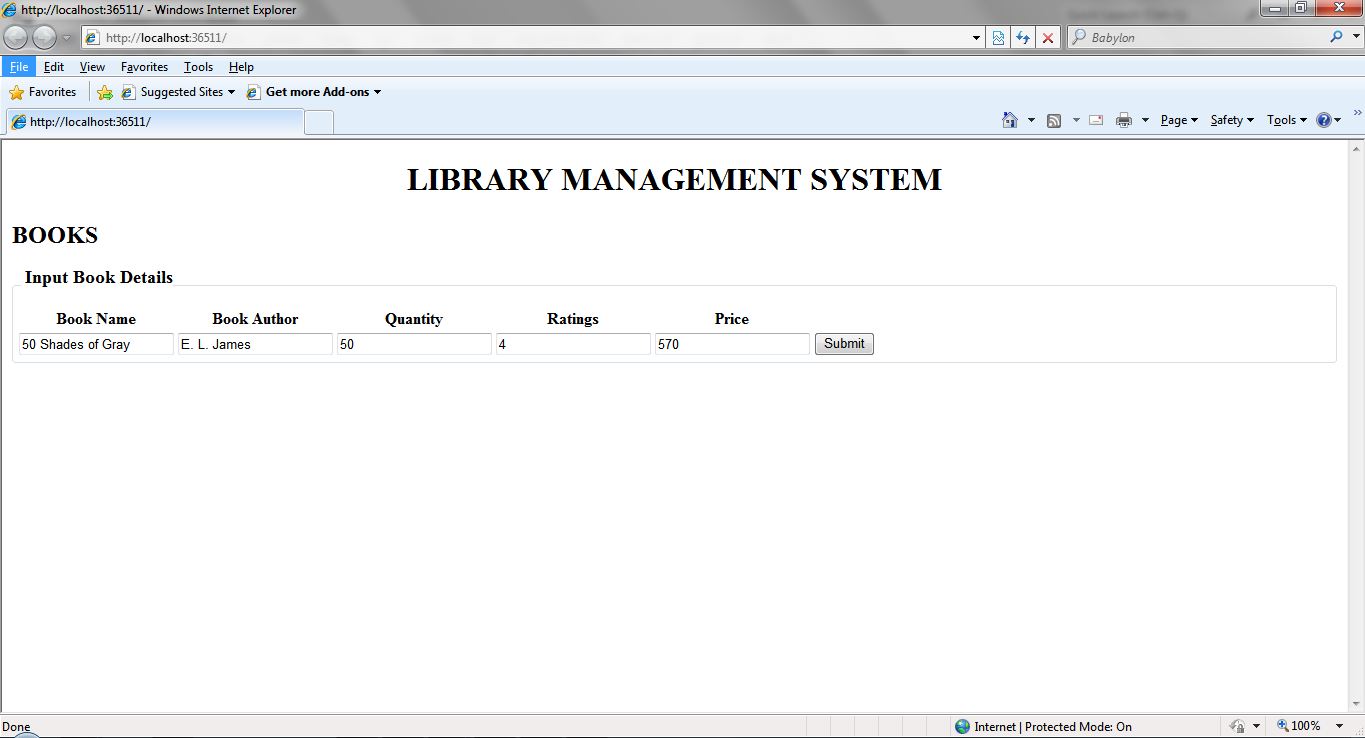
Fig 15: Page showing the book details being displayed
Validation
Server side validation can be done in the model class
present in the Model folder. An appropriate validation message can also be
given in the class library to be displayed in the front end in case of a wrong
entry.
Fig 16: Server side Validation
Coming to an end!
This brings us to an end of our small application that
demonstrates the basic functionality of creating an MVC 4 application. Hoping
that you got an over view of each and every functionality that was used to
create this application. Any queries don’t hesitate to get in touch!
|